What is Exception?
Exception is an event which interrupts the normal flow of the program. Exceptions are mostly related to the application itself. In this blog we will see how we can handle exceptions programmatically and best practices to fallow while handling the exceptions.
Types of Exception?
Exceptions are classified into two types.
1.Checked Exception
These Exception occurs before execution of the program while writing the program code, hence they are examined during compile time. All IOException and InterruptedException exceptions are checked exceptions.
IOException
1.MalformedURLException
2.FileNotFoundException As you see in image below, the complier is throwing the errors before the execution of the program. we need to handle these exceptions before the execution of the program. we are using throws keywork in this example to catch exceptions. We will see further in blog about handling exceptions.
![]() | ![]() |
2.Unchecked Exception
These Exception occurs during run time; hence we need to handle these exceptions carefully while writing the program logic. All run time exceptions are unchecked exception. Let's see some common selenium WebDriver exceptions.
1. TimeoutException : occurs when a command takes more time than the specified or default wait time.
2. SessionNotCreatedException: occurs when WebDriver cannot create a new session.
3. NoSuchSessionException: occurs when we are trying to run any command after driver.quit() or driver.close().
4. ElementNotInteractableException: occurs when element not in a state to interact in spite of present in DOM.
5. ElementNotSelectableException : occurs when element is disabled in spite of present in DOM.
6. ElementNotVisibleException: occurs when element is not visible (HTML type="hidden") in spite of present in DOM.
7. StaleElementReferenceException : occurs when referenced element is no longer present on the DOM page(a reference to component is now stale).
8. NoAlertPresentException: occurs when you switch to no presented alert.
9. NoSuchElementException:occurs if an element could not be found.
10.NoSuchFrameException : occurs if the frame target to be switched to does not exist.
11.InvalidArgumentException :occurs when an argument does not belong to the expected type.
12.InvalidSwitchToTargetException: occurs when the frame or window target to be switched does not exist.
13.WebDriveException: occurs when it tries to perform an action when the webDriver connection is in a closed state.
14.ScreenshotException: occurs when it is not possible to capture a screenshot
15. UnreachableBrowserException: occurs only when the browser is not able to be opened or crashed because of some reason
How to handle the Exception?
Your selenium test should fail, due to failed test cases not because of exceptions that are thrown. if we are getting exception that means we are not handling exceptions logically in our test cases. It's always good technique to write our code in try/catch block. This enables continuation of run even if on test fails due to some exceptions.
1. try-catch A try/catch block is placed around the code which might generate an exception. code within the try/catch block is called protected code. Below is the syntax for using try/catch block.
try{
//protected code
}catch(Exception e){
//code to handle exception
}
2. Multiple catch blocks We can except more than one type of exception from single block of code. We can handle every kind of exceptions using multiple catch blocks. We can use more than two catch blocks there is no limitations on number of catch blocks. Below is the syntax of using multiple catch blocks.
try{
//protected code
}catch(ExceptionType1 e1){
//code to handle ExceptionType1
}
Catch(ExceptionType2 e2){
//code to handle ExceptionType2
}
3.throw/throws
When we are throwing any exception in a method and not handling it, then we need to use throw keyword. throw keyword is used inside the block of code to throw single exception or custom exception.
throws key word is used along with method signature to let caller program know the exceptions that might be thrown by the method while executing the code. Below is the syntax of using throw/throws keywords.
try
{
public void function() throws Exception{
//protected code
}catch(Exception e){
//code to handle Exception
//now throw exception back the system
throw(e)
}
We can provide single or multiple exceptions in the throws clause.
try{
public void function() throws ExceptionType1,ExceptionType2{
//protected code
}catch(ExceptionType1 e1){
//code to handle ExceptionType1
}
Catch(ExceptionType2 e2){
//code to handle ExceptionType2
}
4.Finally Finally, keyword used along with try/catch block code. The finally block code will execute irrespective of occurrence of the exception.
As you see in the below code WebDriver will wait for presence of element located By.id: "test " for 10 seconds and will throw TimeOutException if it's not able to locate with in 10seconds.
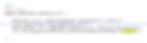
Below is the Exception in console and it will stop further execution of the Test code.

The below code shows how we have handled exceptions using try/catch and finally block .
public void WebDriverException() {
WebDriver driver = WebDriverManager.chromedriver().create();
driver.get("https://www.numpyninja.com/");
try {
// TimeoutException
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(5));
wait.until(ExpectedConditions.presenceOfElementLocated(By.id("test")));
} catch (TimeoutException e) {
System.out.println("Time out Exception occured==> " +e.getMessage());
}catch(Exception e) {
System.out.println("Default Exception occured ");
}
finally {
driver.close();
}
}
The fallowing methods can be used to get the detailed information about the Exceptions.
getMessage(): Displays the description of the Exception.
printStackTrace(): Prints stack trace, name of the Exception, and other useful description.
toString(): Returns a text message describing the exception name and description.
Best Practices to handle Exception
Always predict exception and write code in try/catch/finally block.
Create the custom exception as and when required to make it more readable.
Catch the exception with the exact exception type, for example rather than using Exception use TimeoutException or relevant exception types.
Differentiate between test case errors and coding errors.
use the finally block to clean up the resources.
Conclusion
Exceptions in Selenium cannot be ignored as they disrupt the normal execution of programs.
Programmers can handle standard exceptions using various techniques like Try/catch, Multiple catch blocks, Finally, and others depending upon the requirement of scripts.
Multiple catches help to handle every type of exception with separate block of code.
For analyzing the exceptions in detail, one can also use methods like printStackTrace(), toString(), and getMessage()